Face Recognition and Liveness Check
Verifik offers a Machine Vision solution for Face Recognition and Liveness Detection built with an Edge-AI architecture which eliminates the need for servers.
Comprehensive solution for life detection and face recognition
Verify and authenticate with Liveness Check
Features
Face Recognition and Liveness Check never been this easy
Automated API Onboarding
Send verifications in a simple way using our REST API.
Reduction of the dropout rate
We simplify the user onboarding process with quick and easy identity authentications.
Anti-phishing technology
Real-time face detection, search and comparison.
Seamless Customer Experience
Optimizes incorporation to reduce all types of barriers.
Importance
The importance of
Face Recognition
and Liveness Check
Life Detection is an essential component of the digital identity authentication process. This technology verifies a person's facial features in real time, ensuring authenticity and preventing impersonation attempts or Deepfakes.
Life Detection allows you to detect, recognize and verify faces in images.
These advanced technologies are highly effective: they are easily completed by users, verified quickly and have an excellent ability to detect deepfakes. Therefore, they are very useful tools for Know Your Customer (KYC) and Anti-Money Laundering (AML) processes.
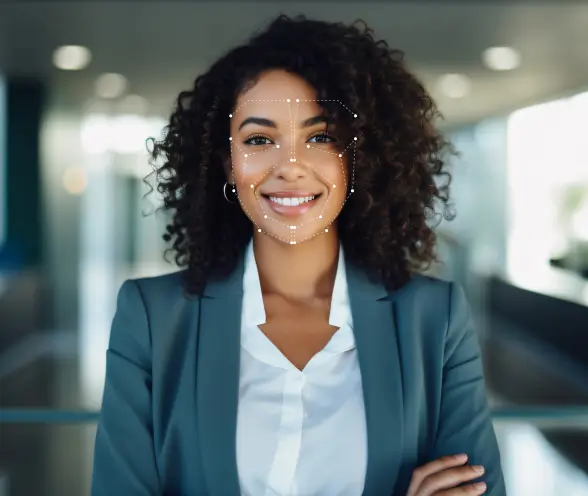
How it works
Code solution
import axios from 'axios';
const options = {
method: 'POST',
url: 'https://api.verifik.co/v2/face-recognition/collections',
headers: {
'Content-Type': 'application/json',
Accept: 'application/json',
Authorization: 'jwt your_token'
},
data: {name: 'string', description: 'string'}
};
try {
const { data } = await axios.request(options);
console.log(data);
} catch (error) {
console.error(error);
}
import requests
url = "https://api.verifik.co/v2/face-recognition/collections"
payload = {
"name": "string",
"description": "string"
}
headers = {
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "JWT eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjbGllbnRJZCI6IjYyM2I2MzE3ZmU1ZmQxNzc0YmU5ZjU2NiIsInYiOjIsInJvbGUiOiJjbGllbnQiLCJjbGllbnRTdWJzY3JpcHRpb25Db2RlIjoiZXh0ZW5kZWRfcGxhbiIsIkpXVFBocmFzZSI6Im5pY29MYUNhZ2FzdGUiLCJleHBpcmVzQXQiOiIyMDIzLTExLTA4IDE0OjA3OjAzIiwiaWF0IjoxNjk2ODYwNDIzfQ.S_6jF7oW-dR-2Gi_xFhOt9gguMV9Z7O2rnN8dP3jvZo"
}
response = requests.post(url, json=payload, headers=headers)
print(response.json())
import Foundation
let headers = [
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "JWT eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjbGllbnRJZCI6IjYyM2I2MzE3ZmU1ZmQxNzc0YmU5ZjU2NiIsInYiOjIsInJvbGUiOiJjbGllbnQiLCJjbGllbnRTdWJzY3JpcHRpb25Db2RlIjoiZXh0ZW5kZWRfcGxhbiIsIkpXVFBocmFzZSI6Im5pY29MYUNhZ2FzdGUiLCJleHBpcmVzQXQiOiIyMDIzLTExLTA4IDE0OjA3OjAzIiwiaWF0IjoxNjk2ODYwNDIzfQ.S_6jF7oW-dR-2Gi_xFhOt9gguMV9Z7O2rnN8dP3jvZo"
]
let parameters = [
"name": "string",
"description": "string"
] as [String : Any]
let postData = try! JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(url: NSURL(string: "https://api.verifik.co/v2/face-recognition/collections")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error as Any)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
<?php
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://api.verifik.co/v2/face-recognition/collections', [
'body' => '{
"name": "string",
"description": "string"
}',
'headers' => [
'Accept' => 'application/json',
'Authorization' => 'JWT eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJjbGllbnRJZCI6IjYyM2I2MzE3ZmU1ZmQxNzc0YmU5ZjU2NiIsInYiOjIsInJvbGUiOiJjbGllbnQiLCJjbGllbnRTdWJzY3JpcHRpb25Db2RlIjoiZXh0ZW5kZWRfcGxhbiIsIkpXVFBocmFzZSI6Im5pY29MYUNhZ2FzdGUiLCJleHBpcmVzQXQiOiIyMDIzLTExLTA4IDE0OjA3OjAzIiwiaWF0IjoxNjk2ODYwNDIzfQ.S_6jF7oW-dR-2Gi_xFhOt9gguMV9Z7O2rnN8dP3jvZo',
'Content-Type' => 'application/json',
],
]);
echo $response->getBody();
import axios from 'axios';
const options = {
method: 'POST',
url: 'https://api.verifik.co/v2/face-recognition/persons/search-live-face',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'jwt your_token'
},
data: {
name: 'Mateo Verifik',
notes: 'Verifik employee',
gender: 'M',
images: ['Base 64 Images'],
min_score: 0.7,
nationality: 'Colombian',
search_mode: 'FAST',
collection_id: 'dac2c81b-12bv-4f19-a6b0-d1a72d55b64b',
date_of_birth: '1995-05-07',
liveness_min_score: 0.5
}
};
try {
const { data } = await axios.request(options);
console.log(data);
} catch (error) {
console.error(error);
}
import requests
url = "https://api.verifik.co/v2/face-recognition/persons/search-live-face"
payload = {
"name": "Mateo Verifik",
"notes": "Verifik employee",
"gender": "M",
"images": ["Base 64 Images"],
"min_score": 0.7,
"nationality": "Colombian",
"search_mode": "FAST",
"collection_id": "dac2c81b-12bv-4f19-a6b0-d1a72d55b64b",
"date_of_birth": "1995-05-07",
"liveness_min_score": 0.5
}
headers = {
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "JWT token"
}
response = requests.post(url, json=payload, headers=headers)
print(response.json())
import Foundation
let headers = [
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "JWT token"
]
let parameters = [
"name": "Mateo Verifik",
"notes": "Verifik employee",
"gender": "M",
"images": ["Base 64 Images"],
"min_score": 0.7,
"nationality": "Colombian",
"search_mode": "FAST",
"collection_id": "dac2c81b-12bv-4f19-a6b0-d1a72d55b64b",
"date_of_birth": "1995-05-07",
"liveness_min_score": 0.5
] as [String : Any]
let postData = try! JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(url: NSURL(string: "https://api.verifik.co/v2/face-recognition/persons/search-live-face")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if let error = error {
print(error)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
<?php
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('POST', 'https://api.verifik.co/v2/face-recognition/collections', [
'body' => '{
"name": "string",
"description": "string"
}',
'headers' => [
'Accept' => 'application/json',
'Authorization' => 'JWT token',
'Content-Type' => 'application/json',
],
]);
echo $response->getBody();
import axios from 'axios';
const options = {
method: 'POST',
url: 'https://api.verifik.co/v2/face-recognition/search',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'jwt your_token'
},
data: {
images: ['base64_encoded_string'],
min_score: 0.7,
search_mode: 'FAST/ACCURATE choose one',
collection_id: 'ID_OF_COLLECTION'
}
};
try {
const { data } = await axios.request(options);
console.log(data);
} catch (error) {
console.error(error);
}
import requests
url = "https://api.verifik.co/v2/face-recognition/search"
payload = {
"images": ["base64_encoded_string"],
"min_score": 0.7,
"search_mode": "FAST/ACCURATE choose one",
"collection_id": "ID_OF_COLLECTION"
}
headers = {
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "JWT token"
}
response = requests.post(url, json=payload, headers=headers)
print(response.json())
import Foundation
let headers = [
"Content-Type": "application/json",
"Accept": "application/json",
"Authorization": "JWT token"
]
let parameters = [
"images": ["base64_encoded_string"],
"min_score": 0.7,
"search_mode": "FAST/ACCURATE choose one",
"collection_id": "ID_OF_COLLECTION"
] as [String: Any]
let postData = try! JSONSerialization.data(withJSONObject: parameters, options: [])
let request = NSMutableURLRequest(
url: NSURL(string: "https://api.verifik.co/v2/face-recognition/search")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0
)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
request.httpBody = postData as Data
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if let error = error {
print(error)
} else {
if let httpResponse = response as? HTTPURLResponse {
print(httpResponse)
}
}
})
dataTask.resume()
<?php
use GuzzleHttp\Client;
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://api.verifik.co/v2/face-recognition/search', [
'body' => '{
"images": [
"base64_encoded_string"
],
"min_score": 0.7,
"search_mode": "FAST/ACCURATE choose one",
"collection_id": "ID_OF_COLLECTION"
}',
'headers' => [
'Accept' => 'application/json',
'Authorization' => 'JWT token',
'Content-Type' => 'application/json',
],
]);
echo $response->getBody();
Solutions
No-Code
A versatile and effective app
Verifik offers a no-code solution for those who prefer a simpler life
dev-ready
Volume discounts available
Verifik offers a coding solution for those who want advanced customization
Technology
Biometric authentication,
done right!
Save on expensive, slow and difficult integrations. In a recent survey, 95% of our customers said they were able to implement it in less than 2 weeks.
Face Recognition
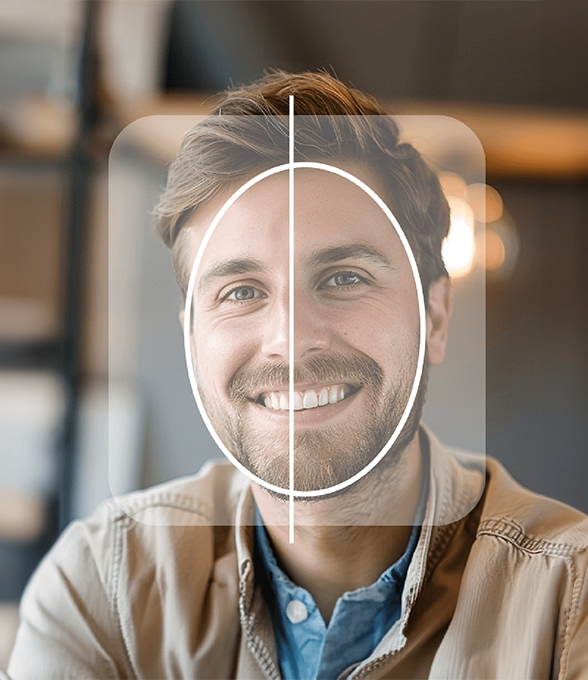

Search and Compare
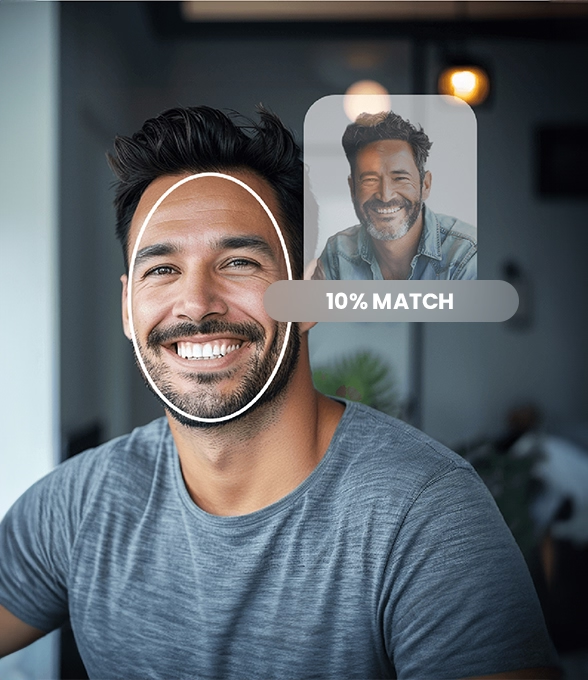
Liveness Detection
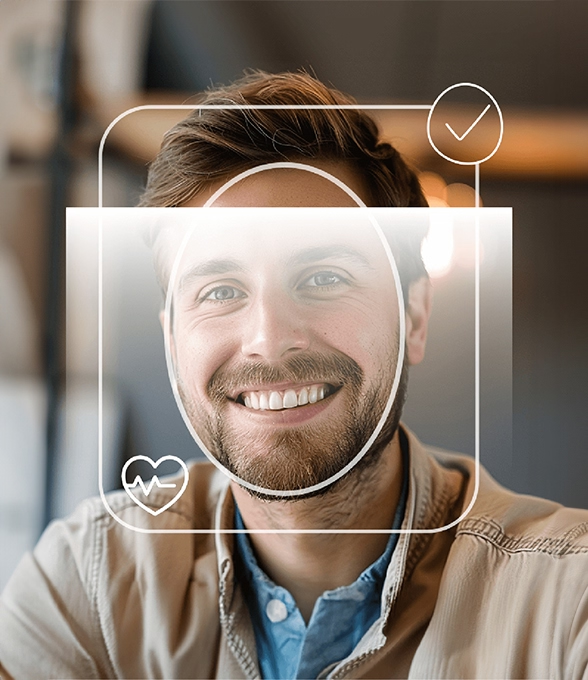
Peer-to-Peer Network
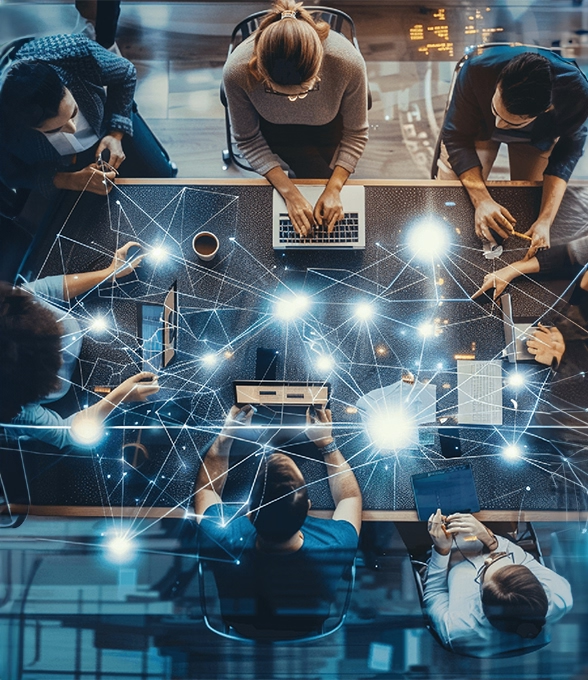
Use Cases
Fintech companies authenticate users with facial recognition during registration and transactions. This not only improves security and reduces the risk of fraud, but also ensures AML and KYC compliance.
Online casinos employ facial recognition to verify players' identities and prevent fraud. This technology ensures that users are who they say they are, protecting both the casino and the players. It also enhances transaction security and personalizes the user experience, creating a secure and reliable environment.
Hotels are using facial recognition to streamline guest check-in, eliminating the need for physical documents and improving security. This technology enables quick identity verification during check-in, personalizing the guest experience and ensuring security standards.
WITH VERIFIK
Plug in, automate and focus on what matters
Products
Choose the best solution for your project with smartENROLL or smartACCESS
App
smartENROLL
A no-code solution for those who prefer a simpler life
- Liveness Detection
- Database Screening
- OCR ID Scanning
App
smartACCESS
A no-code solution for those who prefer a simpler life
- Liveness Detection
- SMS/whatsapp/Email
App
smartENROLL
A no-code solution for those who prefer a simpler life
- Liveness Detection
- Database Screening
- OCR ID Scanning
App
smartACCESS
A no-code solution for those who prefer a simpler life
- Liveness Detection
- SMS/whatsapp/Email
Dev-Ready
smartENROLL
A code solution for those who want advanced customization
- Volume Discounts
- Annual Commitments
Dev-Ready
smartACCESS
A code solution for those who want advanced customization
- Volume Discounts
- Annual Commitments
Dev-Ready
smartENROLL
A code solution for those who want advanced customization
- Volume Discounts
- Annual Commitments
Dev-Ready
smartACCESS
A code solution for those who want advanced customization
- Volume Discounts
- Annual Commitments